Yahoo Search API
Yahoo SERP API provides programmatic access to Yahoo SERPs for developers and businesses. You can use it for various applications like SEO, market research, and competitive analysis.
The SERP API returns 100 SERP Results based on a keyword. The results are specific to the search engine selected. You should be familiar with SERP Types. We provide Web, Image, and News results for Yahoo SERP.
Using Yahoo SERP API, you can get the below result snippets in JSON response.
Recently, We have introduced Image & News API, Where you can perform
search and get result in JSON response
1. TOP STORIES
Top Stories is a feature in Yahoo SERP (Search Engine Results Page) API that displays a list of news articles and stories related to a specific keyword or topic. These articles are curated by Yahoo’s algorithm and are typically based on factors such as the popularity and relevance of the article, the source of the article, and the recency of the publication.
This section is returned by the API as a top_stories array.
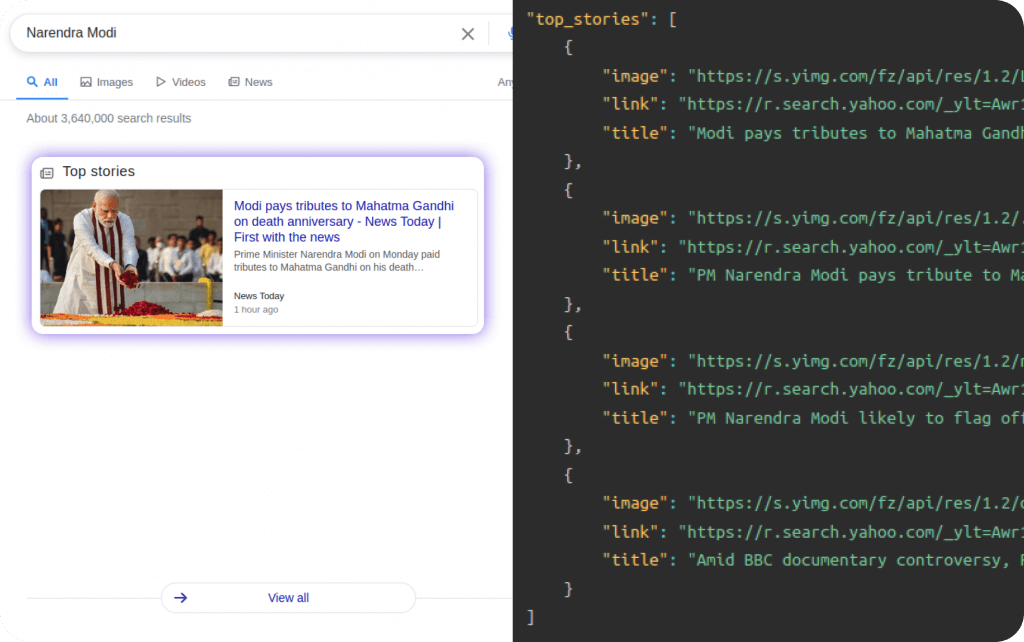
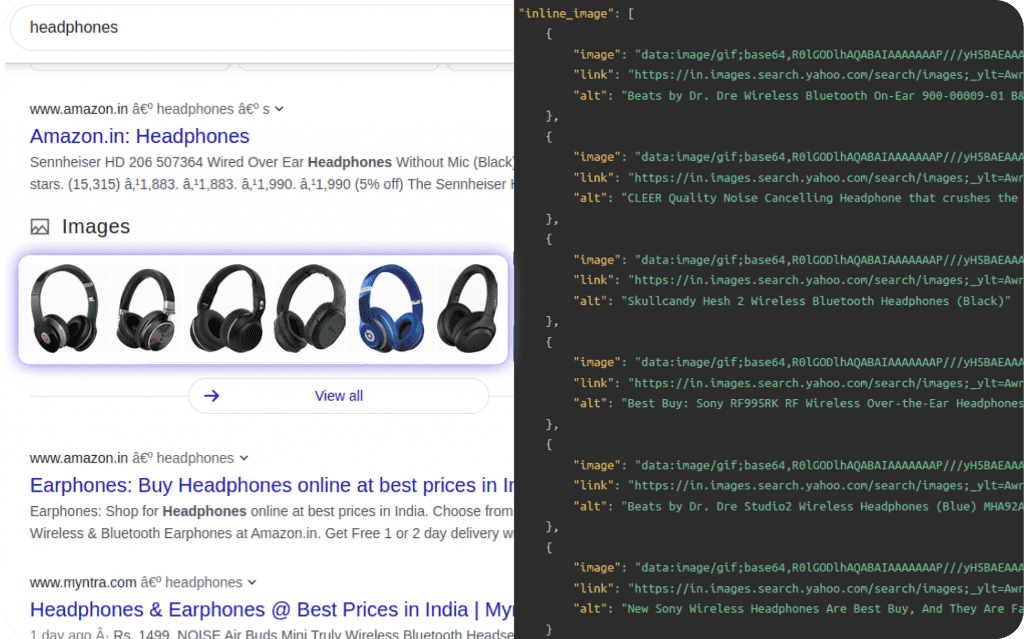
2. INLINE IMAGE
Inline Images is a feature in Yahoo search engine results pages (SERPs) that displays images related to a specific keyword or topic. These images are displayed directly in the search results page, rather than in a separate image search section.
When a user enters a search query related to images, the Inline Images feature in Yahoo search page displays a grid of relevant images along with their source and a brief description of the image. Users can click on any of the images to view a larger version of the image or visit the source website.
When doing a standard web search, images are provided by Yahoo within the search results, these images are returned by the API within an inline_image array.
3. INLINE VIDEOS
Inline Videos is a feature in Yahoo search engine results pages (SERPs) that displays video content related to a specific keyword or topic. These videos are displayed directly in the search results page, rather than in a separate video search section.
When a user enters a search query related to videos, the Inline Videos feature in Yahoo search page displays a list of relevant videos along with their source and a brief description of the video content. Users can click on any of the videos to view the video or visit the source website.
When videos are provided by Yahoo within the search results, these videos are returned by the API within an inline_videos array.
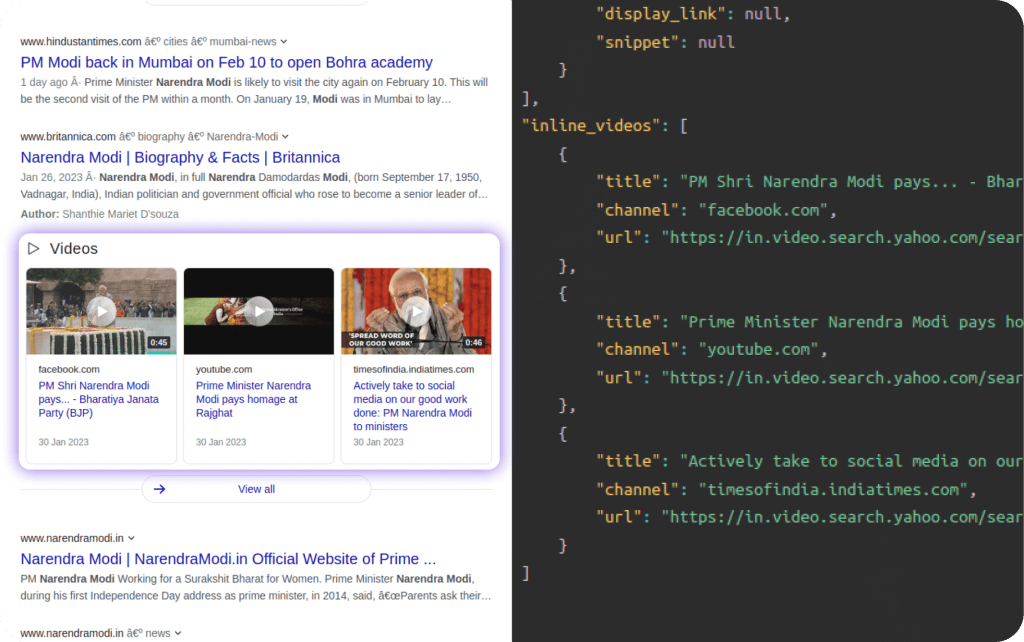
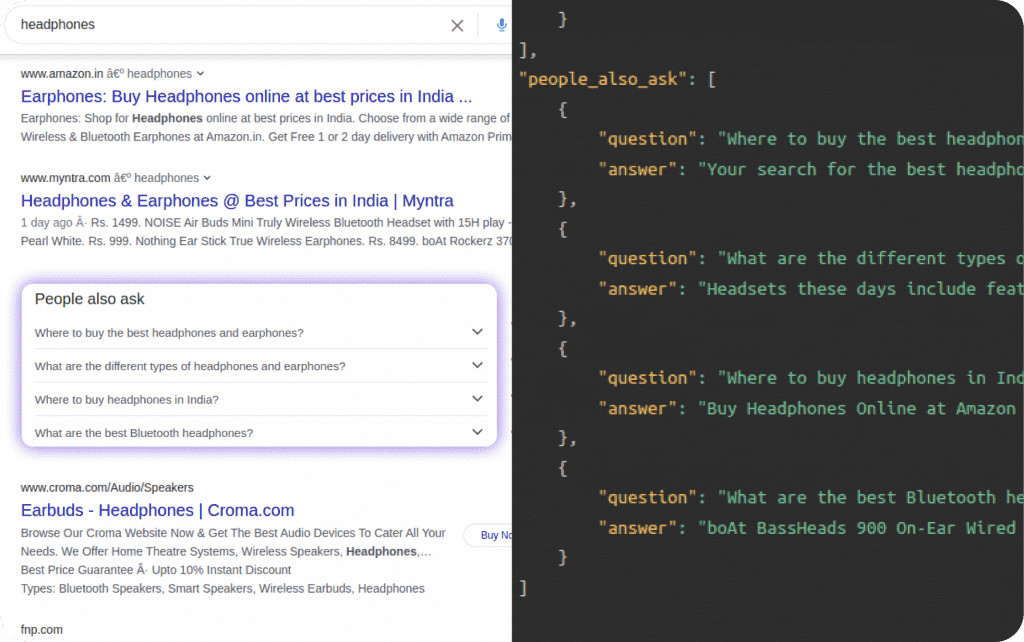
4. PEOPLE ALSO ASK
People also ask is a feature in Yahoo search engine results pages (SERPs) that provides a list of related questions related to a specific search query or topic. This feature aims to help users find more information related to their search query by providing a list of commonly asked questions related to that topic.
When a user enters a search query, the People also ask feature in Yahoo search page displays a list of questions related to that topic in an expandable section. Users can click on any of the questions to view a brief answer or click on “More” to view additional related questions.
For many search queries, Yahoo provides an additional set of frequently asked questions related to the search term. These related questions are returned by the API as a people_also_ask array.
5. KNOWLEDGE CARD
Knowledge Panel is a feature in Yahoo search engine results pages (SERPs) that provides a summary of information related to a specific search query or topic. The knowledge panel is designed to provide quick and easy access to information about a topic without requiring users to click through to other websites or pages.
A Knowledge Graph is returned by Yahoo whenever there is enough information about the search query from Yahoo directly or from informational sources, such as Wikipedia. Knowledge Graphs will be returned in a knowledge_card object by the API.
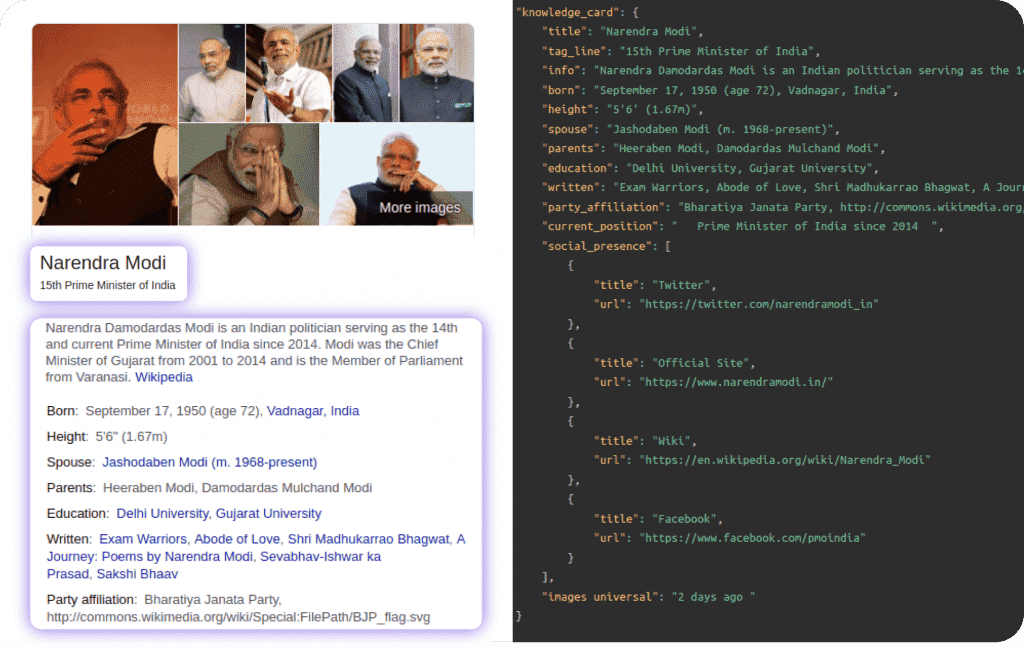
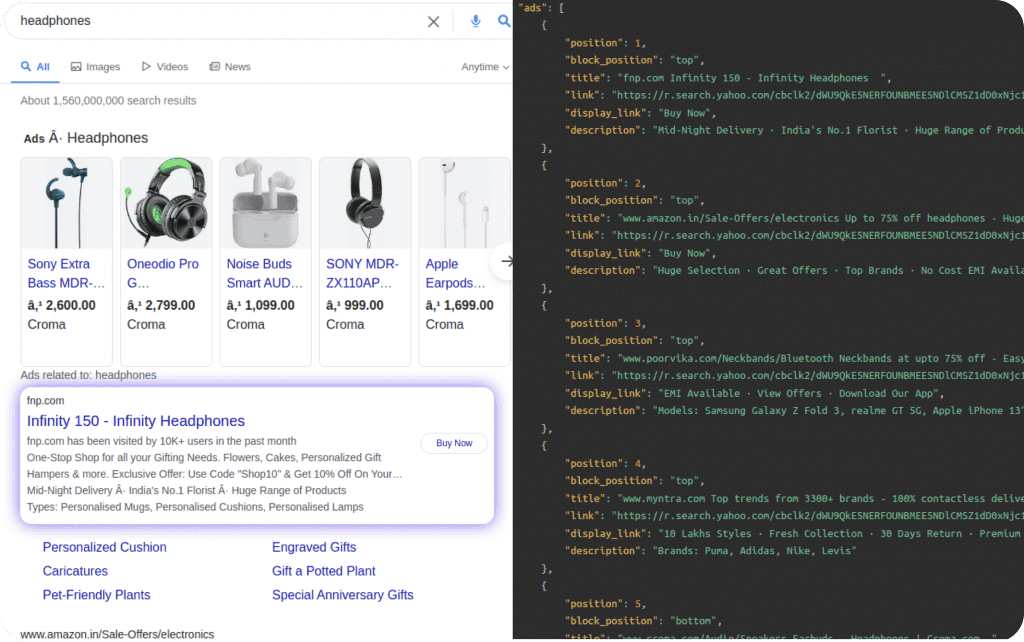
6. ADS
Ads result in Yahoo search pages refer to the paid advertisements displayed at the top and bottom of the search results page. These ads are typically labeled as “sponsored” or “ad” to distinguish them from organic search results.
If Yahoo returns any sponsored ads for your search query, the API response will come with an ads object, which contains all ads in the order they are shown in the search result.
7. ORGANIC
Organic results are the non-advertising search results in Yahoo search engine results pages (SERPs) that appear below the paid advertising results. These results are generated by Yahoo’s algorithm based on the relevance and authority of the website or web page in relation to the user’s search query.
Organic results in Yahoo search page are ranked based on a variety of factors, including the relevance of the content on the website or web page to the user’s search query, the authority and popularity of the website or web page, and the user’s search history and location.
These results are parsed by the API in detail and returned as organic.
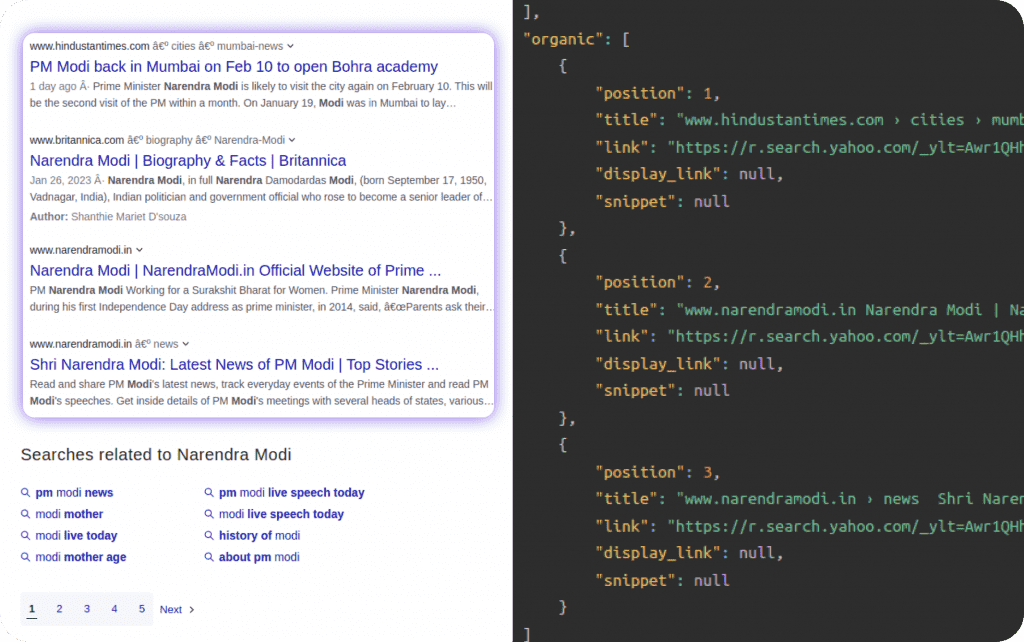
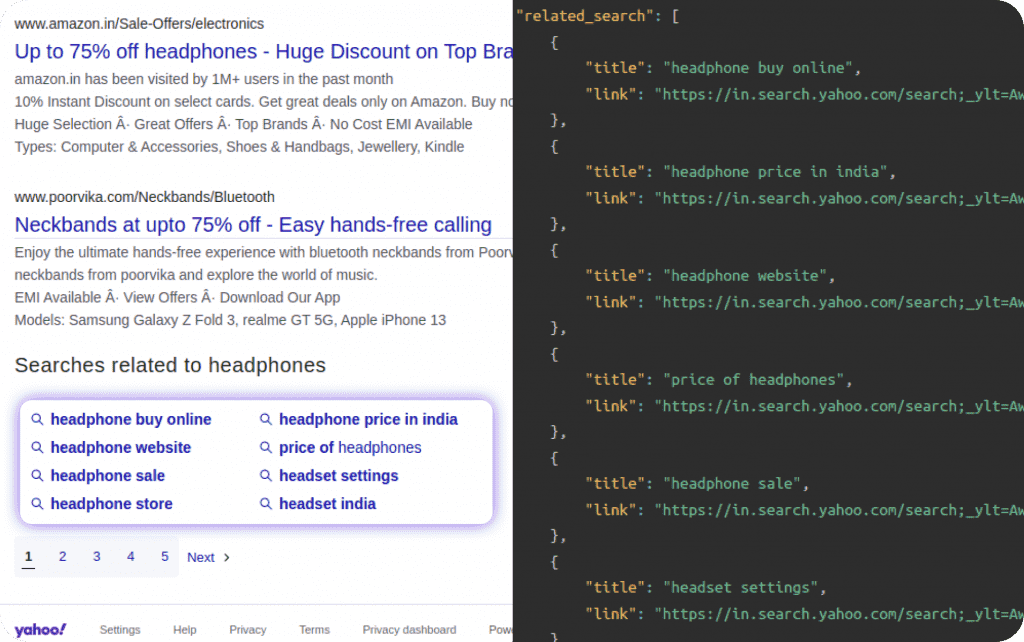
8. RELATED SEARCHES
Related searches can be a helpful tool for finding more specific information related to their search query or discovering new topics related to their interests. From a business perspective, appearing in the related searches section can be valuable for increasing brand awareness and driving traffic to a website or blog. By optimizing website content with relevant keywords and providing high-quality, informative content, businesses can improve the chances of appearing in the related searches section and attracting more traffic to their website or blog.
At the bottom of each search result, Yahoo usually suggests other search queries related to the current query. These suggestions are returned by the API as a related_searches array.
9. NEWS RESULTS
Yahoo News is a dedicated news platform operated by Yahoo, featuring news stories from a variety of sources, including major news organizations, independent journalists, and user-generated content. Yahoo News covers a wide range of topics, including politics, sports, entertainment, finance, technology, and more.
News search on the Yahoo search page can be a valuable tool for staying informed on current events and breaking news related to a specific topic or area of interest.
To retrive a result from Google News, You will need to change your API request param serp_type to news in order to perform a search on news section.
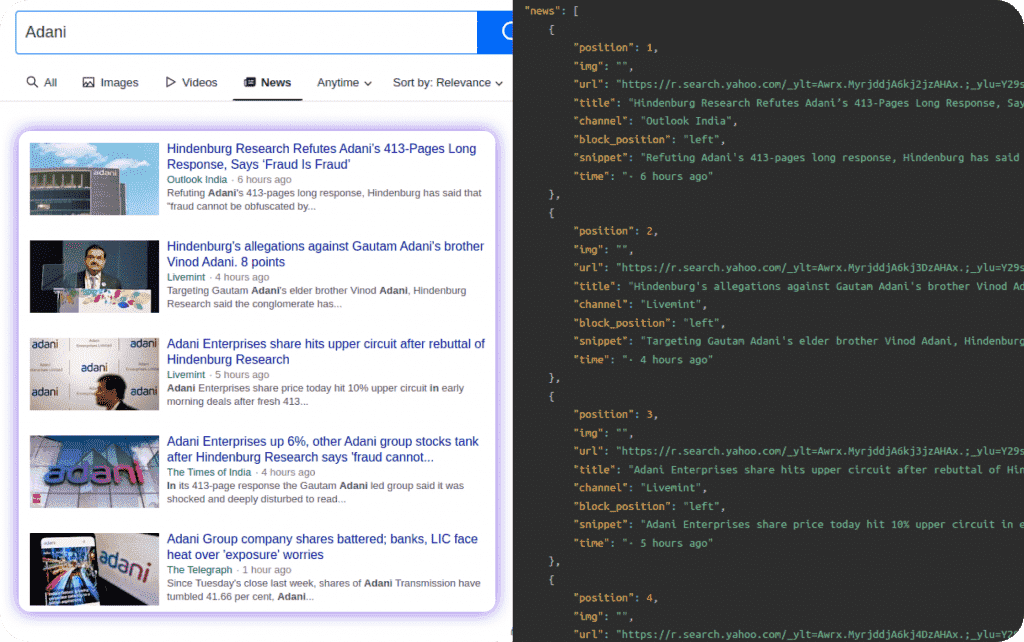
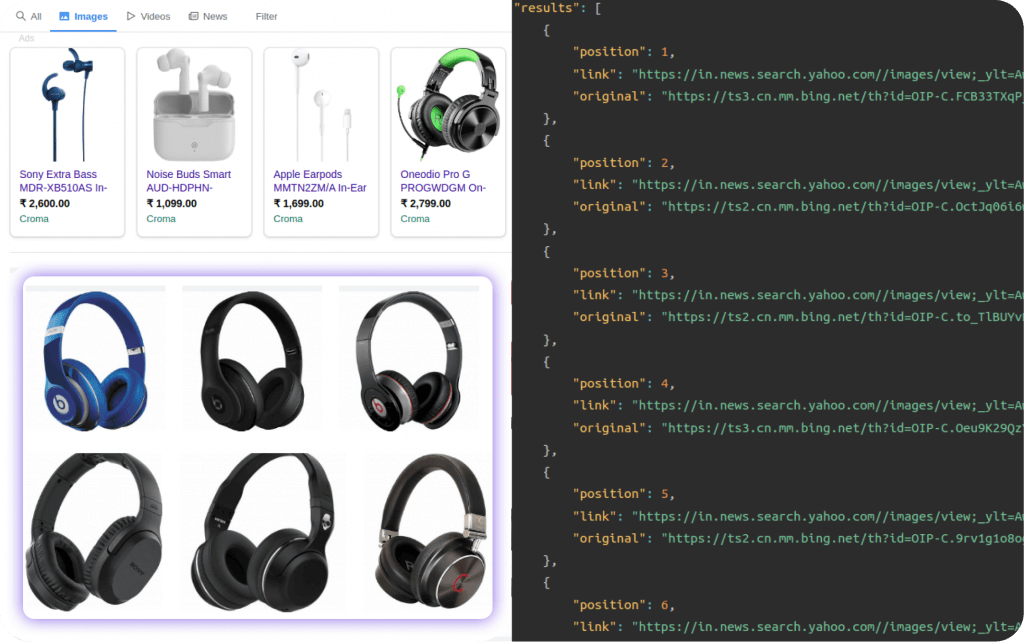
10. IMAGE RESULTS
Yahoo Image search is a dedicated image search platform operated by Yahoo, featuring images from a variety of sources, including stock image websites, social media platforms, and user-generated content. Users can filter their image search results by size, color, type, and other criteria, and can also preview images in a larger format.
Finding and accessing high-quality images related to a specific topic or area of interest. Users can use the images for personal or commercial purposes, depending on the copyright restrictions of the image.
To retrive a result from Google Image, You will need to change your API request param serp_type to image in order to perform a search on image section.
Easy Integration
Select your favoured integration method and copy
the provided code.
curl -X POST \
https://api.serphouse.com/serp/live \
-H 'accept: application/json' \
-H 'authorization: Bearer API_TOKEN' \
-H 'content-type: application/json' \
-d '{
"data": {
"domain":"google.com",
"lang":"en",
"q": "Coffee",
"loc":"Texas,United States",
"device": "desktop",
"serp_type": "web"
}
}'
require 'uri'
require 'net/http'
url = URI("https://api.serphouse.com/serp/live")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request["authorization"] = 'Bearer API_TOKEN'
request.body = '{"data": {
"domain": "google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}}'
response = http.request(request)
puts response.read_body
import requests
url = "https://api.serphouse.com/serp/live"
payload = '{"data": {
"domain": "google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}}'
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "Bearer API_TOKEN"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
var http = require("https");
var options = {
"method": "POST",
"hostname": "https://api.serphouse.com",
"port": null,
"path": "/serp/live",
"headers": {
"accept": "application/json",
"content-type": "application/json",
"authorization": "Bearer API_TOKEN"
}
};
var req = http.request(options, function (res) {
var chunks = [];
res.on("data", function (chunk) {
chunks.push(chunk);
});
res.on("end", function () {
var body = Buffer.concat(chunks);
console.log(body.toString());
});
});
req.write(JSON.stringify({
data: {
domain: 'google.com',
lang: 'en',
q: 'Coffee',
loc: 'Texas,United States',
device: 'desktop',
serp_type: 'web'
}
}));
req.end();
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://api.serphouse.com/serp/live",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => '{"data": {
"domain":"google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}}',
CURLOPT_HTTPHEADER => array(
"accept: application/json",
"authorization: Bearer API_TOKEN",
"content-type: application/json"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
}
else {
echo $response;
}
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, '{"data": {
"domain": "google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"}}
');
Request request = new Request.Builder()
.url("https://api.serphouse.com/serp/live")
.post(body)
.addHeader("accept", "application/json")
.addHeader("content-type", "application/json")
.addHeader("authorization", "Bearer API_TOKEN")
.build();
Response response = client.newCall(request).execute();
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.serphouse.com/serp/live"
payload := strings.NewReader('{"data": {
"domain":"google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"}}
')
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
req.Header.Add("authorization", "Bearer API_TOKEN")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
curl -X POST \
https://api.serphouse.com/serp/live \
-H 'accept: application/json' \
-H 'authorization: Bearer API_TOKEN' \
-H 'content-type: application/json' \
-d '{
"data": {
"domain":"google.com",
"lang":"en",
"q": "Coffee",
"loc":"Texas,United States",
"device": "desktop",
"serp_type": "web"
}
}'
require 'uri'
require 'net/http'
url = URI("https://api.serphouse.com/serp/live")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request["authorization"] = 'Bearer API_TOKEN'
request.body = '{"data": {
"domain": "google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}}'
response = http.request(request)
puts response.read_body
import requests, json
url = "https://api.serphouse.com/serp/live"
payload = json.dumps({
"data": {
"domain": "google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}
})
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "Bearer API_TOKEN"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
var axios = require('axios');
var data = JSON.stringify({
data: {
domain: 'google.com',
lang: 'en',
q: 'Coffee',
loc: 'Texas,United States',
device: 'desktop',
serp_type: 'web'
}
});
var config = {
"method": "POST",
"url": "https://api.serphouse.com/serp/live",
"headers": {
"content-type": "application/json",
"authorization": "Bearer API_TOKEN"
},
data : data
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error){
console.log(error);
});
$client = new GuzzleHttp\Client();
$headers= [
'Authorization' => 'Bearer API_TOKEN',
'Content-Type' => 'Application/json',
];
$body = '{
"data" : {
"domain": "google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}}';
$request = new GuzzleHttp\Psr7\Request('POST','https://api.serphouse.com/serp/live', $headers, $body);
$res = $client->sendAsync($request)->wait();
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("application/json");
JSONObject jsonObject = new JSONObject();
JSONObject data = new JSONObject();
data.put("domain", "google.com");
data.put("lang", "en");
data.put("q", "Coffee");
data.put("loc", "Texas,United States");
data.put("device", "desktop");
data.put("serp_type", "web");
jsonObject.put("data", data);
String bodyJson = jsonObject.toString();
RequestBody body = RequestBody.create(mediaType, bodyJson);
Request request = new Request.Builder()
.url("https://api.serphouse.com/serp/live")
.method("POST", body)
.addHeader("content-type", "application/json")
.addHeader("authorization", "Bearer API_TOKEN")
.build();
Response response = client.newCall(request).execute();
System.out.println(response.body().string());
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.serphouse.com/serp/live"
payload := strings.NewReader(`{"data": {
"domain":"google.com",
"lang": "en",
"q": "Coffee",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"}}
`)
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
req.Header.Add("authorization", "Bearer API_TOKEN")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
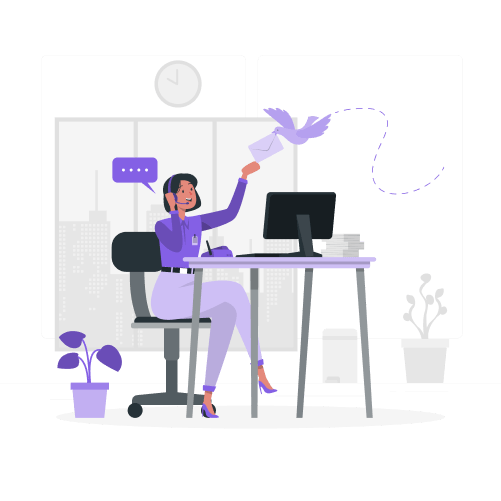
You may contact us from here for any concern.