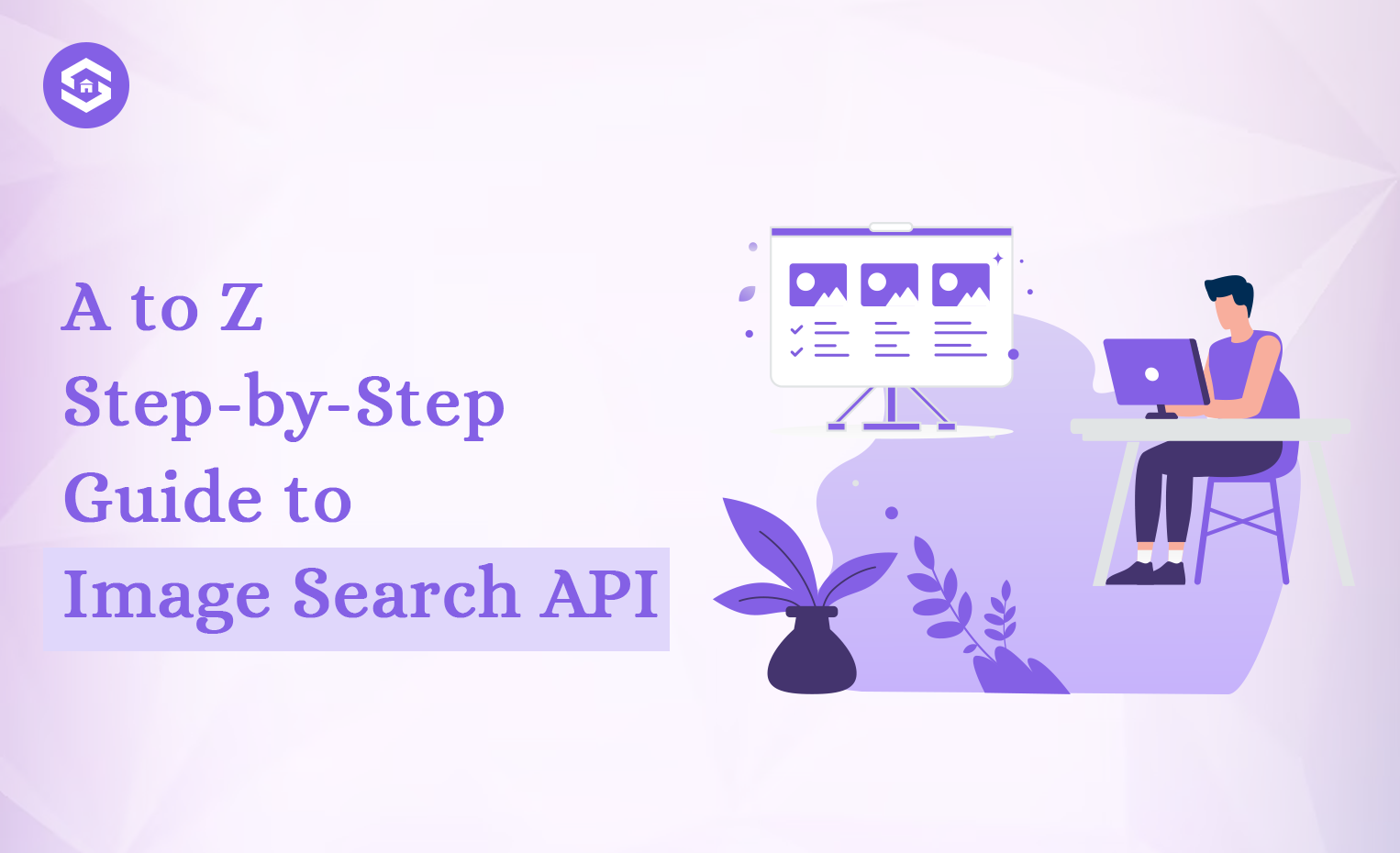
Image Search APIs, like Google’s, let developers fetch images from the internet through code.
These APIs simplify and automate image searches, making it easier to add this features to apps, websites, and services.
Benefits & Use Cases of Image Search API
Benefits
- Rich Visual Content
- Time Efficiency
- Personalization
- Content Discovery
Use Cases
- E-commerce
- Content Creation
- Visual Recognition
- Travel & Tourism
- Education
- Social Media
Prerequisites for Getting Started
In this blog, we exclusively discuss the Google Image API. To get started, you’ll need to:
Create a Google Account:
Sign up for a Google account to access developer tools and set up a Custom Search Engine (CSE).
Set Up a Custom Search Engine (CSE):
Generate a CSE on the Google Custom Search Engine page, configuring it for image search. Make a note of your CSE ID.
Obtain an API Key:
Get an API key from the Google Developers Console to authenticate your API requests. Be sure to enable the Custom Search JSON API for your project.
Once you have these prerequisites, you can make API requests and integrate image search functionality into your applications and websites using the Image retrieval API.
Read More: how to set up Your development environment for Obtaining API Key
You’re all set! With a Google account, a configured Custom Search Engine for images, and an API key, you’re ready to request image search results.
Making API Requests
Constructing API Request URLs
To search for images using the Image Scraper API, you must construct a URL that includes your API key, Custom Search Engine (CSE) ID, and the search query. Here’s how to build the API request URL:

- Replace YOUR_API_KEY with your actual API key obtained from the Google Developers Console.
- Replace YOUR_CSE_ID with the Custom Search Engine ID you created for image search.
- Replace SEARCH_QUERY with the query you want to search for (e.g., “beautiful landscapes”).
The searchType=image parameter specifies that you want to perform an image search.
Sending HTTP Requests
You can use various programming languages and libraries to send HTTP GET requests to the constructed API URL.
Below is an example in Python using the popular requests library:
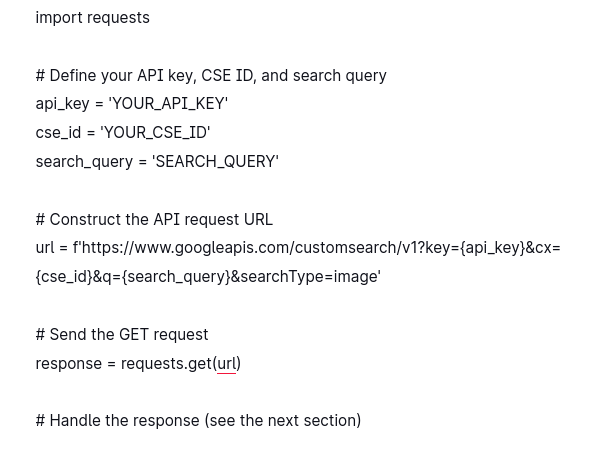
In this example, we import the requests library, define the necessary parameters, construct the URL, and send a GET request to the Google Image API.
Handling API Responses
The Google Image Search API responds with search results in JSON format. Using your chosen programming language, you can parse and extract data from the API response. Here’s how you can access image search results in Python:
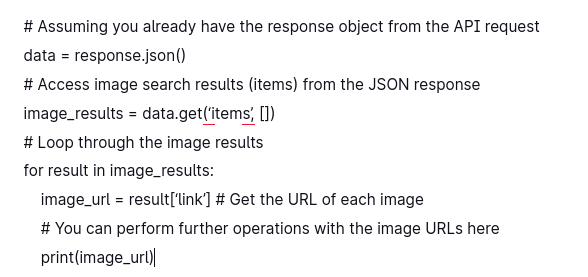
In this code, we extract image URLs by parsing the JSON response using .json() and accessing the ‘items’ key.
Your specific implementation may vary depending on your programming language and libraries.
Be sure to handle errors, pagination if necessary, and any extra data you need from the API response to meet your project’s needs.
Understanding the API Response
Parsing JSON Data
The Google Image scraper responds with JSON (JavaScript Object Notation) format search results. To work with this data, you’ll need to parse it using your chosen programming language. Most programming languages provide libraries or functions for parsing JSON data. Here’s an example in Python:
Code:
# Assuming you have the API response stored in the ‘response’ variable
data = response.json()
Accessing Image Search Results
You can access the image search results once you’ve parsed the JSON response. The results are typically found in the ‘items’ key of the JSON response. You can extract them like this in Python:
Code:
image_results = data.get(‘items’, [])
This code snippet retrieves the list of image results or an empty list if there are no results.
Extracting Metadata
Each image search result in the API response contains metadata about the image. Common metadata includes the image URL, image title, and image snippet. Here’s how you can extract this information in Python:
Code:
for result in image_results:
image_url = result[‘link’] # URL of the image
image_title = result[‘title’] # Title of the image
image_snippet = result[‘snippet’] # Snippet or description of the image
# You can use or store this metadata as needed for your application
Advanced API Usage
Pagination and Result Limits
By default, the Google Image retrieval API may limit the number of results returned in a single response. To access additional results, you can use pagination by specifying the start parameter in your API request.
For example, to get the second page of results with 10 results per page, set the start to 11:
Filtering and Refining Searches
To refine your image searches, you can use advanced search operators in your query. For example, you can specify image size, colour, file type, and more. These operators help narrow down your search to specific criteria. Here’s an example of a refined query:
Code:
SEARCH_QUERY site:wikipedia.org filetype:png
This query searches for images related to SEARCH_QUERY but only from the wikipedia.org domain and is limited to PNG file types.
Handling Rate Limits
Stay within rate limits when using Google’s API services. Monitor usage in the Google Developers Console and refer to the official documentation for current limits.
Advanced use of the Google Image Search API includes optimizing queries, pagination, and rate limit awareness for efficient image searches.
Billing and Usage Considerations
Billing Information
When using the Google Image Search scraper, be mindful of possible charges based on your usage.
Costs may arise depending on your request frequency, query types, and result quantities, as Google’s billing considers these factors
Check the Google Cloud Pricing page for detailed information about pricing, billing plans, and any free usage limits that may be available.
You may Also Check: SERPHouse Pricing.
Usage Limits and Quotas
Google’s APIs have usage limits to prevent misuse and promote fairness.
These limits depend on your billing plan, API type, and your requests.
It’s essential to read the documentation, monitor your usage, and avoid surpassing your quota, as it could lead to rate limiting or extra fees.
Compliance with Terms of Service
Ensure that your application or service complies with Google’s Terms of Service. Consider any usage restrictions, data usage policies, and attribution requirements.
Failure to comply with the Terms of Service can suspend or terminate your API access.
Sample Code and Programming Languages
We offer code samples in different languages for the Google Image Search API.
These snippets show how to use the API, process responses, and add image search to your apps.
Pick the language that suits your project best.
Python Example
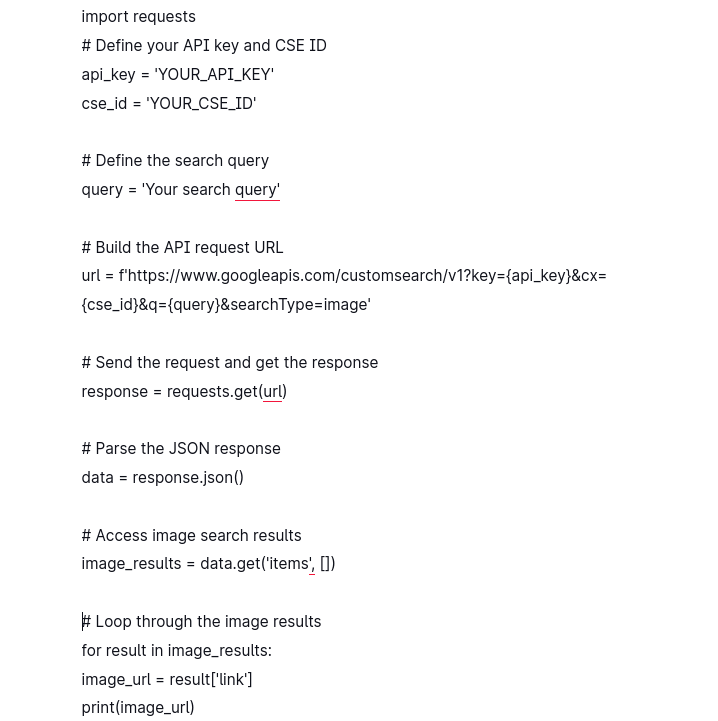
JavaScript Example
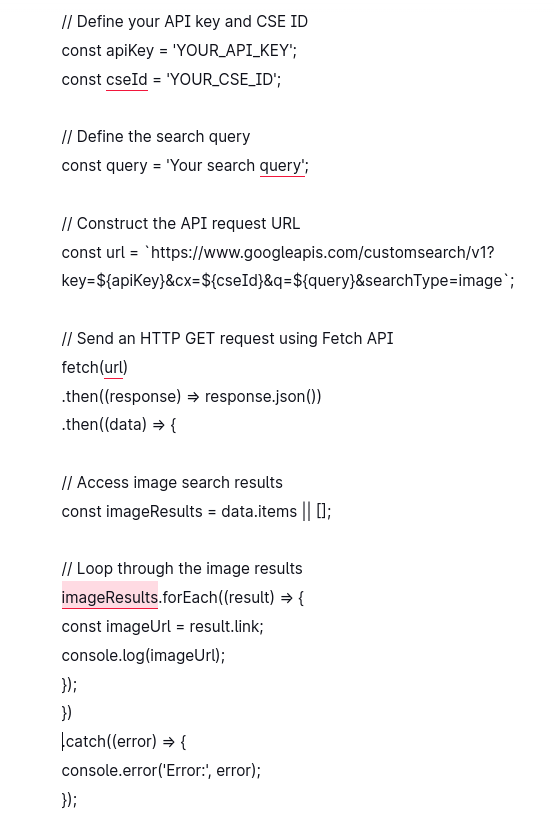
Integrating the API into Your Application
These examples help you begin with the Google Image Search API.
Customize as needed by replacing ‘YOUR_API_KEY’ and ‘YOUR_CSE_ID’. Check the API docs for advanced features.
Use any programming language; it’s compatible with many.
Best Practices for Image Search
Optimizing Search Queries
Enhance search results with precise keywords, filters, and advanced operators. Experiment with settings for better outcomes.
Displaying Search Results
Prioritize layout, responsiveness, and user experience when showing search results. Arrange images neatly and include important metadata.
Handling Image Thumbnails
For fast loading and better user experience, use suitable image formats and sizes for thumbnails in search results.
Troubleshooting
Common Issues and Solutions
API Key Errors:
Ensure your API key is correctly configured and included in your API requests. Double-check the key’s permissions and restrictions.
Rate Limit Exceeded:
Monitor your API usage and avoid exceeding rate limits. Implement rate limiting on your end to prevent issues.
Empty or Incomplete Responses:
Handle cases where the API response may be open or missing expected data gracefully.
Incorrect Search Queries:
Verify that your search queries are correctly formatted and relevant to your application’s needs.
Frequently Asked Questions
Focus on product-related keywords, use filters for product categories and provide high-quality image previews.
Google Image Search API typically supports many image formats, including JPEG, PNG, GIF, and more.
It’s important to respect copyright and licensing. Ensure you have the necessary rights or permissions for the images you display.
Experiment with different search queries, filters, and sorting options to prioritize the most relevant results.
Review the API documentation, double-check your API key and request parameters, and consider contacting Google’s support if the issue persists.
Conclusion and Next Steps
Summary of Key Takeaways
In this guide, we’ll explore the Google Image Search API, covering the basics, advanced features, billing, and best practices for developers and businesses.
Learn how to set up your environment, and manage pagination, filters, and rate limits.
Discover tips for optimizing search queries and handling image thumbnails. We’ll also address common issues and FAQs for a smooth experience with the API.
Links to Official Documentation
To dive deeper into the world of image search and API development, consider exploring the following resources:
- Google Custom Search JSON API Documentation
- Google Developers Console Documentation
- There are online communities and forums for discussions and problem-solving.
Keeping Up with API Updates
Stay updated on Google API changes by visiting the Google Developers website regularly.
Subscribe to relevant newsletters for notifications.
Also, watch the Google Cloud blog for updates on Google’s APIs and services.
With this knowledge, you’re equipped to harness the power of image search in your applications and adapt to evolving technologies and best practices in the field. Happy coding!