Google Local Business Result API
The Google Local Places Results API is a tool that allows users to gather information from a Google Local Places search.
When you use SERPHouse with this API, you unlock the capability to extract and make sense of the data. SERPHouse is an interpreter, helping you understand and utilize the information effectively.
It’s like having a language translator for the digital world, ensuring you can easily access and work with the details you need from your Google Places search results.
- position
- title
- rating
- reviews_count
- type
- address
- open_state
JSON structure overview
{
"local_pack": [
{
"position": 1,
"title": "Modern Market Eatery",
"rating": "4.1",
"reviews_count": "(629)",
"type": "Health Food",
"address": "401 Congress Ave. · In Frost Bank Tower",
"open_state": "Closed \u22c5 Opens 10\u202fAM "
}
]
}
Results for: restaurants
https://api.serphouse.com/serp/live?q=restaurants&domain=google.com&lang=en&device=desktop&serp_type=web&loc=Austin,Texas,United States&loc_id=1026201&verbatim=0&gfilter=0&page=1&num_result=100
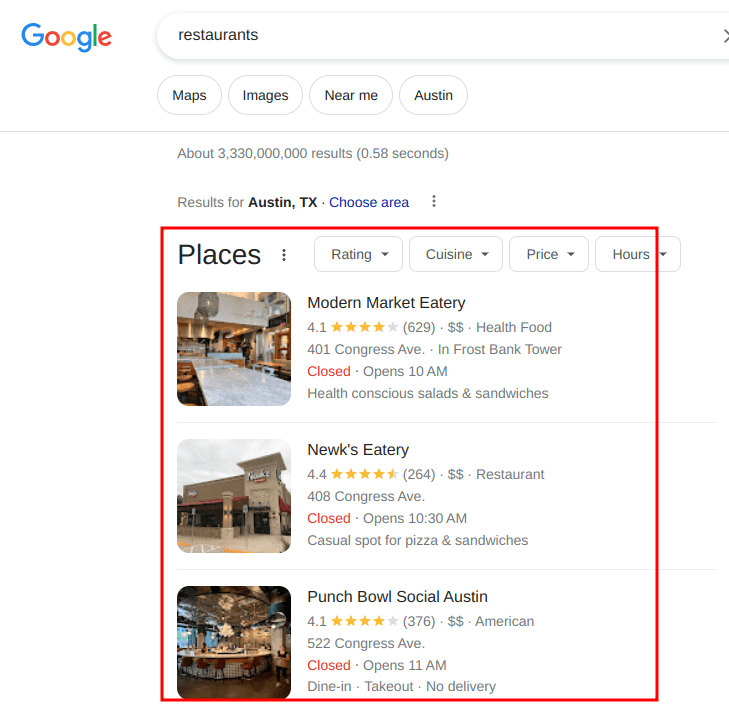
Curl
Ruby
Python
Node.js
PHP
Java
GO
Curl
curl -X POST \
https://api.serphouse.com/serp/live \
-H 'accept: application/json' \
-H 'authorization: Bearer API_TOKEN' \
-H 'content-type: application/json' \
-d '{
"data": {
"domain":"google.com",
"lang":"en",
"q": "restaurants",
"loc":"Texas,United States",
"device": "desktop",
"serp_type": "web"
}
}'
Ruby
require 'uri'
require 'net/http'
url = URI("https://api.serphouse.com/serp/live")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request["authorization"] = 'Bearer API_TOKEN'
request.body = '{"data": {
"domain": "google.com",
"lang": "en",
"q": "restaurants",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}}'
response = http.request(request)
puts response.read_body
Python
import requests, json
url = "https://api.serphouse.com/serp/live"
payload = json.dumps({
"data": {
"domain": "google.com",
"lang": "en",
"q": "restaurants",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}
})
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "Bearer API_TOKEN"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
Node.js
var axios = require('axios');
var data = JSON.stringify({
data: {
domain: 'google.com',
lang: 'en',
q: 'restaurants',
loc: 'Texas,United States',
device: 'desktop',
serp_type: 'web'
}
});
var config = {
"method": "POST",
"url": "https://api.serphouse.com/serp/live",
"headers": {
"content-type": "application/json",
"authorization": "Bearer API_TOKEN"
},
data : data
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error){
console.log(error);
});
PHP
$client = new GuzzleHttp\Client();
$headers= [
'Authorization' => 'Bearer API_TOKEN',
'Content-Type' => 'Application/json',
];
$body = '{
"data" : {
"domain": "google.com",
"lang": "en",
"q": "restaurants",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"
}}';
$request = new GuzzleHttp\Psr7\Request('POST','https://api.serphouse.com/serp/live', $headers, $body);
$res = $client->sendAsync($request)->wait();
Java
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("application/json");
JSONObject jsonObject = new JSONObject();
JSONObject data = new JSONObject();
data.put("domain", "google.com");
data.put("lang", "en");
data.put("q", "restaurants");
data.put("loc", "Texas,United States");
data.put("device", "desktop");
data.put("serp_type", "web");
jsonObject.put("data", data);
String bodyJson = jsonObject.toString();
RequestBody body = RequestBody.create(mediaType, bodyJson);
Request request = new Request.Builder()
.url("https://api.serphouse.com/serp/live")
.method("POST", body)
.addHeader("content-type", "application/json")
.addHeader("authorization", "Bearer API_TOKEN")
.build();
Response response = client.newCall(request).execute();
System.out.println(response.body().string());
GO
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.serphouse.com/serp/live"
payload := strings.NewReader(`{"data": {
"domain":"google.com",
"lang": "en",
"q": "restaurants",
"loc": "Texas,United States",
"device": "desktop",
"serp_type": "web"}}
`)
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
req.Header.Add("authorization", "Bearer API_TOKEN")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
{
...
"local_pack": [
{
"position": 1,
"title": "Modern Market Eatery",
"rating": "4.1",
"reviews_count": "(629)",
"type": "Health Food",
"address": "401 Congress Ave. · In Frost Bank Tower",
"open_state": "Closed \u22c5 Opens 10\u202fAM "
},
{
"position": 2,
"title": "Newk's Eatery",
"rating": "4.4",
"reviews_count": "(264)",
"type": "Restaurant",
"address": "408 Congress Ave.",
"open_state": "Closed \u22c5 Opens 10:30\u202fAM "
}
]
...
}
Results for: headphone near me on mobile
https://api.serphouse.com/serp/live?q=headphone+near+me&domain=google.com&lang=en&device=mobile&serp_type=web&loc=Austin,Texas,United States&loc_id=1026201&verbatim=0&gfilter=0&page=1&num_result=100
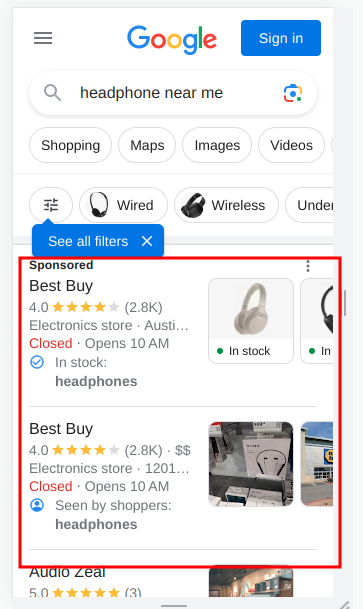
Curl
Ruby
Python
Node.js
PHP
Java
GO
Curl
curl -X POST \
https://api.serphouse.com/serp/live \
-H 'accept: application/json' \
-H 'authorization: Bearer API_TOKEN' \
-H 'content-type: application/json' \
-d '{
"data": {
"domain":"google.com",
"lang":"en",
"q": "headphone near me",
"loc":"Texas,United States",
"device": "mobile",
"serp_type": "web"
}
}'
Ruby
require 'uri'
require 'net/http'
url = URI("https://api.serphouse.com/serp/live")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["accept"] = 'application/json'
request["content-type"] = 'application/json'
request["authorization"] = 'Bearer API_TOKEN'
request.body = '{"data": {
"domain": "google.com",
"lang": "en",
"q": "headphone near me",
"loc": "Texas,United States",
"device": "mobile",
"serp_type": "web"
}}'
response = http.request(request)
puts response.read_body
Python
import requests, json
url = "https://api.serphouse.com/serp/live"
payload = json.dumps({
"data": {
"domain": "google.com",
"lang": "en",
"q": "headphone near me",
"loc": "Texas,United States",
"device": "mobile",
"serp_type": "web"
}
})
headers = {
'accept': "application/json",
'content-type': "application/json",
'authorization': "Bearer API_TOKEN"
}
response = requests.request("POST", url, data=payload, headers=headers)
print(response.text)
Node.js
var axios = require('axios');
var data = JSON.stringify({
data: {
domain: 'google.com',
lang: 'en',
q: 'headphone near me',
loc: 'Texas,United States',
device: 'mobile',
serp_type: 'web'
}
});
var config = {
"method": "POST",
"url": "https://api.serphouse.com/serp/live",
"headers": {
"content-type": "application/json",
"authorization": "Bearer API_TOKEN"
},
data : data
};
axios(config)
.then(function (response) {
console.log(JSON.stringify(response.data));
})
.catch(function (error){
console.log(error);
});
PHP
$client = new GuzzleHttp\Client();
$headers= [
'Authorization' => 'Bearer API_TOKEN',
'Content-Type' => 'Application/json',
];
$body = '{
"data" : {
"domain": "google.com",
"lang": "en",
"q": "headphone near me",
"loc": "Texas,United States",
"device": "mobile",
"serp_type": "web"
}}';
$request = new GuzzleHttp\Psr7\Request('POST','https://api.serphouse.com/serp/live', $headers, $body);
$res = $client->sendAsync($request)->wait();
Java
OkHttpClient client = new OkHttpClient().newBuilder().build();
MediaType mediaType = MediaType.parse("application/json");
JSONObject jsonObject = new JSONObject();
JSONObject data = new JSONObject();
data.put("domain", "google.com");
data.put("lang", "en");
data.put("q", "headphone near me");
data.put("loc", "Texas,United States");
data.put("device", "mobile");
data.put("serp_type", "web");
jsonObject.put("data", data);
String bodyJson = jsonObject.toString();
RequestBody body = RequestBody.create(mediaType, bodyJson);
Request request = new Request.Builder()
.url("https://api.serphouse.com/serp/live")
.method("POST", body)
.addHeader("content-type", "application/json")
.addHeader("authorization", "Bearer API_TOKEN")
.build();
Response response = client.newCall(request).execute();
System.out.println(response.body().string());
GO
package main
import (
"fmt"
"strings"
"net/http"
"io/ioutil"
)
func main() {
url := "https://api.serphouse.com/serp/live"
payload := strings.NewReader(`{"data": {
"domain":"google.com",
"lang": "en",
"q": "headphone near me",
"loc": "Texas,United States",
"device": "mobile",
"serp_type": "web"}}
`)
req, _ := http.NewRequest("POST", url, payload)
req.Header.Add("accept", "application/json")
req.Header.Add("content-type", "application/json")
req.Header.Add("authorization", "Bearer API_TOKEN")
res, _ := http.DefaultClient.Do(req)
defer res.Body.Close()
body, _ := ioutil.ReadAll(res.Body)
fmt.Println(res)
fmt.Println(string(body))
}
{
...
"local_pack": [
{
"position": 1,
"title": "Best Buy ",
"rating": "4.0",
"reviews_count": "(2.8K)",
"type": "Electronics store ",
"address": "Austin, TX",
"open_state": "Closed \u22c5 Opens 10\u202fAM "
},
{
"position": 2,
"title": "Best Buy ",
"rating": "4.0",
"reviews_count": "(2.8K)",
"type": "Electronics store ",
"address": "1201 Barbara Jordan Blvd Ste 100",
"open_state": "Closed \u22c5 Opens 10\u202fAM "
},
{
"position": 3,
"title": "Audio Zeal ",
"rating": "5.0",
"reviews_count": "(3)",
"type": "Home audio store ",
"address": "2110 S Lamar Blvd",
"open_state": "Closed \u22c5 Opens 9\u202fAM "
},
]
...
}
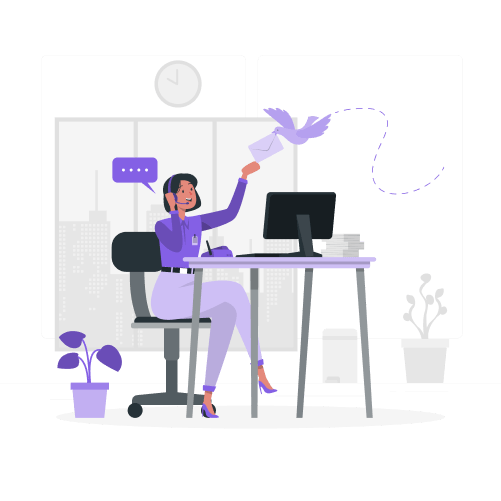
Get in touch with us!
You may contact us from here for any concern.